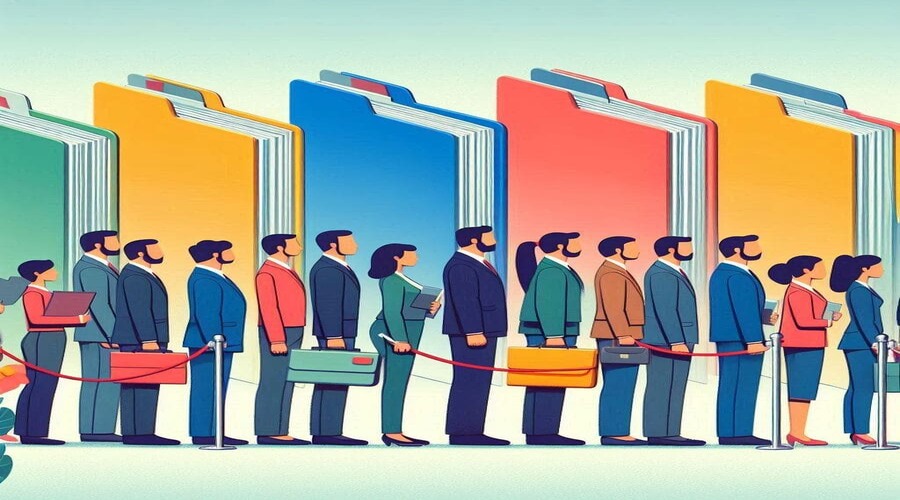
Why do you need Laravel Queue?
When we build a web application, some tasks take too much time such as emailing new users, reading CSV files, and many more. Laravel provides a queued job. Queue job is running such all processed in the background.
Step 1: Configure Queue Connection
Ensure your .env file is configured to use a queue connection other than Sync. You can use the database for simplicity. By default, Laravel uses the Sync driver, which executes queue jobs immediately.
QUEUE_CONNECTION = database
Step 2: Queue Configuration
A database table is required to hold the jobs with a database connection. A database table is required to hold the jobs with the database queue driver.
php artisan queue:table
After migration is created, migrate your database using the following command:
php artisan migrate
Step 3: Creating Jobs
By default, all queued jobs are stored in the app/jobs directory. To create new jobs, run the following artisan command into your terminal.
php artisan make:job NewUserWelcomeMail
This command create App\Jobs\NewUserWelcomeMail class which extends the Illuminate\Contracts\Queue\ShouldQueue; interface. Indicating to Laravel that the job should be pushed onto the queue to run asynchronously.
<?php
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Facades\Mail;
use App\Mail\WelcomeMail;
class NewUserWelcomeMail implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
protected $user;
/**
* Create a new job instance.
*/
public function __construct($user)
{
$this->user = $user;
}
/**
* Execute the job.
*/
public function handle(): void
{
Mail::to($this->user->email)->send(new Welcomemail($this->user));
}
}
Step 4: Create a Mailable
php artisan make:mail WelcomeMail
This will create a new mailable class under app/Mail directory, named WelcomeMail.php. Open this file and define the email content and settings
Step 5: Dispatch Queue Jobs
Here we create a user a user controller for creating users. It will look like the one below.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Jobs\NewUserWelcomeMail;
use App\Models\User;
use Illuminate\Support\Facades\Hash;
class UserController extends Controller
{
public function create(Request $request){
// create a user
$user = User::create([
"name"=> 'krunal',
"email" => 'krunal3g@gmail.com',
"password" => Hash::make(123456)
]);
// dispatch your queue job
dispatch(new NewUserWelcomeMail($user));
echo 'Mail sent successfully';
}
}
Step 6: Running Queue Jobs
Laravel includes an Artisan command that will start a queue worker and process new jobs as they are pushed onto the queue.
php artisan queue:work
You can use the queue:listen command. You don't need to manually restart the worker to reload the updated code or reset the application state.
php artisan queue:listen
Good Luck 🙂
* Please Don't Spam Here. All the Comments are Reviewed by Admin.